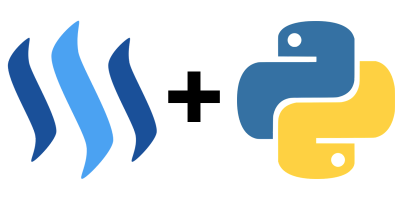
<p dir="auto">This tutorial is part of a series where different aspects of programming with <code>steem-python are explained. Links to the other tutorials can be found in the curriculum section below. This part is a direct continuation of <a href="https://steemit.com/utopian-io/@steempytutorials/part-23-retrieve-and-process-full-blocks-from-the-steem-blockchain" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 23: Retrieve And Process Full Blocks From The Steem Blockchain and will go into more detail following <code>operation types: <code>transfer, <code>transfer_to_vesting, <code>withdraw_vesting and <code>convert.
<hr />
<h4>Repository
<p dir="auto"><span><a href="https://github.com/steemit/steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">https://github.com/steemit/steem-python
<h4>What will I learn
<ul>
<li>How to access data in a operation
<li>What is a transfer?
<li>What is a transfer_to_vesting
<li>What is a withdraw_vesting
<li>What is a convert?
<h4>Requirements
<ul>
<li>Python3.6
<li><code>steem-python
<h4>Difficulty
<ul>
<li>basic
<hr />
<h3>Tutorial
<h4>Setup
<p dir="auto">Download the files from <a href="https://github.com/amosbastian/steempy-tutorials/tree/master/part_24" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Github. There 2 are files <code>get_blocks.py which contains the code. The main file takes 3 arguments from the command line which sets the <code>starting_block, <code>block_count and what <code>operation to filter for.
<p dir="auto">Run scripts as following:<br />
<code>> python get_block.py 22224731 10 transfer
<h4>How to access data in a operation
<p dir="auto">The previous tutorial went into great detail how to extract <code>operations from <code>blocks. There are 19 different <code>operations in total and each has a different structure. However, the data for the <code>operation is always stored in the same spot.
<pre><code>{
'ref_block_num': 8004,
'ref_block_prefix': 1744181707,
'expiration': '2018-05-07T15:29:54',
'operations': [
['vote', {
'voter': 'acehjaya',
'author': 'zunaofficial79',
'permlink': 'kabut-pagi',
'weight': 10000
}]
],
'extensions': [],
'signatures': ['1f205086670c27365738697f0bc7cfde8b6e976608dc12b0d391b2b85ad7870a002313be4b09a358c30010be2a09bebacead866ba180afc348ce40c70566f4ed88']
}
<p dir="auto"><br /><br />
Under <code>operations is an array, the first index is the <code>operation type and the second index is a <code>json table with the <code>operation data.
<pre><code>if transaction['operations'][0][0] == self.tag:
self.process_operation(transaction['operations']
[0][1],
self.tag)
<p dir="auto">Filter for the required operation type and process the <code>json data. For this example tutorial a different <code>class is made for each <code>operation type which stores the data in respective <code>variables and has a <code>print function. These classes can be found in the <code>operations.py file.
<h4>What is a transfer?
<p dir="auto">Every <code>transfer of either <code>Steem or <code>SBD between two different user accounts is a <code>transfer operation. This operation has 4 variables. <code>from is the account that sends the funds, <code>to the receiving account, <code>amount the valuation and which currency, and optionally <code>memo. In which messages can be added.
<pre><code>{
'from': 'yusril-steem',
'to': 'postpromoter',
'amount': '5.000 SBD',
'memo': 'https://steemit.com/life/@yusril-steem/our-challenges-and-trials-in-life'
}
<p dir="auto"><br /><br />
By using a <code>class to process the <code>operation all the <code>variables can be neatly kept and additional <code>functions can be added as well. Like the <code>print_operation() which allows for easy printing to the terminal. A separate <code>class is made for each of the <code>operations discussed in this tutorial.
<pre><code>class Transfer():
def __init__(self, operation, block, timestamp):
self.account = operation['from']
self.to = operation['to']
self.amount = operation['amount']
self.memo = operation['memo']
self.block = block
self.timestamp = timestamp
def print_operation(self):
print('\nBlock:', self.block, self.timestamp)
print('Operation: transfer')
print('From:', self.account)
print('To:', self.to)
print('Amount:', self.amount)
print('Memo:', self.memo)
print('')
<p dir="auto"><br /><br />
Additional data that normally falls outside the scope of the <code>operation can also be stored in the <code>class. Like the <code>block in which the <code>operation took place and the timestamp of the <code>operation.
<h4>What is a transfer_to_vesting
<p dir="auto">A <code>power up of <code>Steem by a user is known as a <code>transfer_to_vesting and contains 3 <code>variables. <code>from the user account itself, <code>to the receiving account and <code>amount the amount being powered up. This <code>operation locks up the <code>Steem for 13 weeks.
<pre><code>{
'from': 'flxsupport',
'to': 'ltcil',
'amount': '0.719 STEEM'
}
<p dir="auto"><br />
<h4>What is a withdraw_vesting
<p dir="auto">Opposite to a <code>transfer_to_vesting is a <code>withdraw_vesting. When a user initiates a <code>power down the amount of <code>steem power will be powered down in 13 weekly increments. <code>Vested steem power is displayed in <code>VESTS and has to be converted to <code>Steem based on the current conversion rate. This is outside the scope of this tutorial but can be found <a href="https://steemit.com/utopian-io/@steempytutorials/part-22-calculate-account-value-based-on-current-market-prices-via-api" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">here. This <code>operation holds 2 different <code>variables. <code>account the user account and <code>vesting_shares the amount of <code>VESTS to be converted to <code>Steem at the time of the operation.
<pre><code>{
'account': 'bazimir',
'vesting_shares': '44671.906021 VESTS'
}
<p dir="auto"><br />
<h4>What is a convert?
<p dir="auto">It is possible to convert <code>SBD to <code>Steem on the <code>internal market. This <code>operation assumes that the value of <code>SBD is at $1.0, which may not be true. Always check the <code>external markets before doing such an <code>operation. After 3 days the <code>operation matures and the user will receive the <code>Steem. This <code>operation holds 3 different <code>variables: <code>amount the amount of <code>SBD to be converted, <code>owner the account initiating the conversion and <code>requestid the id for the system to perform the conversion after 3 days.
<pre><code>{
'amount': '5000.0 SBD',
'owner': 'steemit',
'requestid': 1467592156
}
<p dir="auto"><br />
<h4>Running the script
<p dir="auto">Running the script will print the <code>operations, for which the filter has been set, to the terminal starting from the <code>starting_block for <code>block_count amount of <code>blocks. A <code>class object is made for the <code>operation and the build in <code>print_operation() function is called. The data of the <code>operation is printed to the terminal with the additional information of the <code>block in which the <code>operation is contained and the <code>timestamp of the <code>operation.
<pre><code>python get_blocks.py 22224731 10 transfer
Booted
Connected to: https://rpc.buildteam.io
Block: 22224731
Block: 22224731 2018-05-07T15:20:03
Operation: transfer
From: hottopic
To: jgullinese
Amount: 0.001 SBD
Memo: Hello Friend, Your post will be more popular and you will find new friends.We provide "Resteem upvote and promo" service.Resteem to 18.000+ Follower,Min 45+ Upvote.Send 1 SBD or 1 STEEM to @hottopic (URL as memo) Service Active
Block: 22224731 2018-05-07T15:20:03
Operation: transfer
From: minnowbooster
To: msena
Amount: 0.001 SBD
Memo: You got an upgoat that will be done by bycz,greece-lover,hemanthoj,percygeorge,bigdave2250,mexresorts,wanxlol,nickmorphew,thauerbyi,blackbergeron,aziz96,lovlu,utube,lowestdefinition. We detected an open value of 0.0$, worth 0.001 SBD in send and refunding that! Request-Id: 1176288
...
...
...
<h4>Curriculum
<h5>Set up:
<ul>
<li><a href="https://steemit.com/utopian-io/@amosbastian/how-to-install-steem-python-the-official-steem-library-for-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 0: How To Install Steem-python, The Official Steem Library For Python
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-1-how-to-configure-the-steempy-cli-wallet-and-upvote-an-article-with-steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 1: How To Configure The Steempy CLI Wallet And Upvote An Article With Steem-Python
<h5>Filtering
<ul>
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-2-how-to-stream-and-filter-the-blockchain-using-steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 2: How To Stream And Filter The Blockchain Using Steem-Python
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-6-how-to-automatically-reply-to-mentions-using-steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 6: How To Automatically Reply To Mentions Using Steem-Python
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-23-retrieve-and-process-full-blocks-from-the-steem-blockchain" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 23: Part 23: Retrieve And Process Full Blocks From The Steem Blockchain
<h5>Voting
<ul>
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-3-creating-a-dynamic-upvote-bot-that-runs-24-7-first-weekly-challenge-3-steem-prize-pool" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 3: Creating A Dynamic Autovoter That Runs 24/7
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-4-how-to-follow-a-voting-trail-using-steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 4: How To Follow A Voting Trail Using Steem-Python
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-8-how-to-create-your-own-upvote-bot-using-steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 8: How To Create Your Own Upvote Bot Using Steem-Python
<h5>Posting
<ul>
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-5-post-an-article-directly-to-the-steem-blockchain-and-automatically-buy-upvotes-from-upvote-bots" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 5: Post An Article Directly To The Steem Blockchain And Automatically Buy Upvotes From Upvote Bots
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-7-how-to-schedule-posts-and-manually-upvote-posts-for-a-variable-voting-weight-with-steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 7: How To Schedule Posts And Manually Upvote Posts For A Variable Voting Weight With Steem-Python
<h6>Constructing
<ul>
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-10-use-urls-to-retrieve-post-data-and-construct-a-dynamic-post-with-steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 10: Use Urls To Retrieve Post Data And Construct A Dynamic Post With Steem-Python
<h5>Rewards
<ul>
<li><a href="https://steemit.com/utopian-io/@steempytutorials/how-to-calculate-a-post-s-total-rewards-using-steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 9: How To Calculate A Post's Total Rewards Using Steem-Python
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-12-how-to-estimate-curation-rewards" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 12: How To Estimate Curation Rewards Using Steem-Python
<li><a href="https://steemit.com/utopian-io/@steempytutorials/how-to-estimate-all-rewards-in-last-n-days-using-steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 14: How To Estimate All Rewards In Last N Days Using Steem-Python
<h5>Transfers
<ul>
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-11-how-to-build-a-list-of-transfers-and-broadcast-these-in-one-transaction-with-steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 11: How To Build A List Of Transfers And Broadcast These In One Transaction With Steem-Python
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-13-upvote-posts-in-batches-based-on-current-voting-power-with-steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 13: Upvote Posts In Batches Based On Current Voting Power With Steem-Python
<h5>Account Analysis
<ul>
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-15-how-to-check-if-an-account-is-following-back-and-retrieve-mutual-followers-following-between-two-accounts" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 15: How To Check If An Account Is Following Back And Retrieve Mutual Followers/Following Between Two Accounts
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-16-how-to-analyse-a-user-s-vote-history-in-a-specific-time-period-using-steem-python" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 16: How To Analyse A User's Vote History In A Specific Time Period Using Steem-Python
<li><a href="https://steemit.com/utopian-io/@steempytutorials/part-18-how-to-analyse-an-account-s-resteemers" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Part 18: How To Analyse An Account's Resteemers Using Steem-Python
<hr />
<p dir="auto">The code for this tutorial can be found on <a href="https://github.com/amosbastian/steempy-tutorials/tree/master/part_24" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">GitHub!
<p dir="auto"><span>This tutorial was written by <a href="/@juliank">@juliank.
I thank you for your contribution. Here are my thoughts on your post;
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Chat with us on Discord.
[utopian-moderator]Need help? Write a ticket on https://support.utopian.io/.
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!Hey @steempytutorials
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Thank you for your very good tutorial !