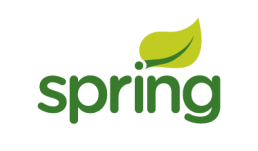
<p dir="auto">Source<a href="https://www.javatpoint.com/images/spimages/spring1.png" target="_blank" rel="nofollow noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Here
<h4>What Will I Learn?
<p dir="auto"><span>In this tutorial, we will learn about how we can develop Rest web API or Services using <a href="/@restcontroller">@RestController annotation in Spring framework and how you can call the web services using ARC Client
<ul>
<li>Rest Web services
<li>Get JSON data from Rest web Services using ARC or Web Browser
<h4>Requirements
<ul>
<li>Eclipse IDE (Juno or above)
<li>JDK 1.7 or above
<li>Tomcat Server 7 or above
<li>Spring and jackson jar files
<li>Web Browser Chrome or Firefox
<h4>Difficulty
<ul>
<li>Basic
<h4>Tutorial Contents
<ul>
<li>Application Configuration wit Spring Framework
<p dir="auto"><span>Now we configure the web application with sping framework to creating <a href="/@restcontroller">@restController
<ol>
<li>configuring web.xml file<br />
First of all paste all jar files into lib folder<br />
Here we configure web.xml file to run application application
<pre><code><?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<servlet>
<servlet-name>spring</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/config/spring-servlet.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>spring</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file></welcome-file>
</welcome-file-list>
</web-app>
<p dir="auto"><img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012856/sl4tmx5pf01oo2jkqofb.png" alt="Screenshot (103).png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012856/sl4tmx5pf01oo2jkqofb.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012856/sl4tmx5pf01oo2jkqofb.png 2x" />
<ol>
<li>configuring spring-servlet.xml<br />
Basic configuration of databse, view resolver and sessionfactory
<pre><code><?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-3.0.xsd">
<pre><code><context:property-placeholder location="classpath:resources/database.properties" />
<context:component-scan base-package="com.blog" />
<pre><code> <bean id="jspViewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="viewClass" value="org.springframework.web.servlet.view.JstlView" />
<property name="prefix" value="/" />
<property name="suffix" value=".jsp" />
</bean>
```
<pre><code><bean id="sessionFactory" class="org.springframework.orm.hibernate3.annotation.AnnotationSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="annotatedClasses">
<list>
<value>com.blog.model.Users</value>
</list>
</property>
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">${hibernate.dialect}</prop>
<prop key="hibernate.show_sql">${hibernate.show_sql}</prop>
<prop key="hibernate.hbm2ddl.auto">${hibernate.hbm2ddl.auto}</prop>
</props>
</property>
</bean>
<pre><code>
</beans>
<p dir="auto"><img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012832/wq4boushyywgq6yoplrb.png" alt="Screenshot (104).png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012832/wq4boushyywgq6yoplrb.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012832/wq4boushyywgq6yoplrb.png 2x" />
<ul>
<li>Creating RestController<br />
Here we create RestControler java file and write code for populating list and converlist from ArrayList to json and this controler return json object string
<pre><code>package com.blog.restwebservice;
import java.io.IOException;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.List;
import org.codehaus.jackson.JsonGenerationException;
import org.codehaus.jackson.map.JsonMappingException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import com.blog.bean.QuestionsBean;
import beans.ConvertDateFormat;
import beans.ListToJsonObject;
import beans.Sudent;
import com.blog.model.Questions;
import com.blog.service.QuestionsService;
@RestController
@RequestMapping("/yayayaa")
public class RestWebServiceController {
@Autowired(required=false)
private QuestionsService questionsService;
ListToJsonObject listtojson = new ListToJsonObject();
ConvertDateFormat fmt = new ConvertDateFormat();
@RequestMapping(method = RequestMethod.GET)
public @ResponseBody String listQuestionsss() throws JsonGenerationException, JsonMappingException, IOException, ParseException {
List<Sudent> list = listofStudents();
String json = new ObjectMapper().writeValueAsString(list);
return json;
}
List<Sudent> list = new ArrayList<Sudent>();
public List<Sudent> listofStudents()
{
list.add(new Sudent(1,20,"Rahul","Whatever","rahul@gmail.com"));
list.add(new Sudent(2,21,"Ramesh","Whatever","ramesh@gmail.com"));
list.add(new Sudent(3,22,"Shyam","Whatever","shyam@gmail.com"));
list.add(new Sudent(4,23,"Ram","Whatever","ram@gmail.com"));
list.add(new Sudent(5,24,"Krishna","Whatever","krishna@gmail.com"));
list.add(new Sudent(6,25,"Jacop","Whatever","jacop@gmail.com"));
return list;
}
}
<p dir="auto"><img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012892/bhzpvotg0rsp48hef9sv.png" alt="Screenshot (106).png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012892/bhzpvotg0rsp48hef9sv.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012892/bhzpvotg0rsp48hef9sv.png 2x" />
<h3>Result
<ul>
<li><p dir="auto">Lets run the project using tomcat server call the controller using ARC Client<br />
<img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012493/gazows1dozstbkjuewtv.png" alt="Screenshot (108).png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012493/gazows1dozstbkjuewtv.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012493/gazows1dozstbkjuewtv.png 2x" />
<li><p dir="auto">run on web browser<br />
<img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012563/lfotzpxpatt59jyv3xqf.png" alt="Screenshot (107).png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012563/lfotzpxpatt59jyv3xqf.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012563/lfotzpxpatt59jyv3xqf.png 2x" />
<li><p dir="auto">parse retuned JSON data with json parser<br />
<img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012668/temdozmcxfwl8xaud9xv.png" alt="Screenshot (109).png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012668/temdozmcxfwl8xaud9xv.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1516012668/temdozmcxfwl8xaud9xv.png 2x" />
<p dir="auto">It's Done !<br />
Congratulations ! we successfully developed Rest Web Service.
<h4>Thank You !
<p dir="auto">Share from the core of my heart
<p dir="auto"><br /><hr /><em>Posted on <a href="https://utopian.io/utopian-io/@ravik2492/how-to-develop-rest-web-service-using-restcontroller-spring-framework" target="_blank" rel="nofollow noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Utopian.io - Rewarding Open Source Contributors<hr /><p>
Great work. Make a complete tutorial
Thank you ! 2 hours to complete the work and half hour to write tutorial
Your contribution cannot be approved because it does not follow the Utopian Rules.
Rest controller is not open_ Source.
You can contact us on Discord.
[utopian-moderator]