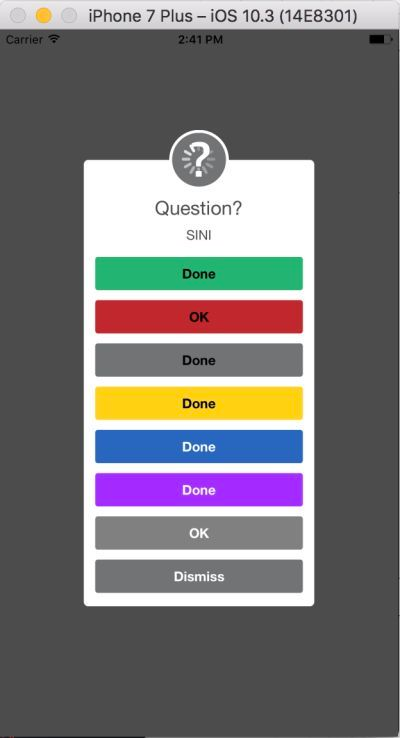
<h4>What Will I Learn?
<ul>
<li>Integrate SCLAlertView to iOS project
<li>Install Cocoapods
<li>Import SCLAlertView library
<li>Implement SCLAlertView to display alert in various ways like show error, show warning, show success messages, etc
<h4>Requirements
<ul>
<li>Xcode
<li>Install Cocoapods
<li>Understanding of Objective-C language in iOS development
<h4>Difficulty
<ul>
<li>Basic
<h4>Tutorial Contents
<p dir="auto"><b>Integrate the library via Cocoapods<br />
Make sure you have installed Cocoapods. Add this line at the Podfile <code>pod 'SCLAlertView-Objective-C'. This is the entire Podfile.
<pre><code># Uncomment the next line to define a global platform for your project
# platform :ios, '9.0'
target 'PopUpDemo' do
# Uncomment the next line if you're using Swift or would like to use dynamic frameworks
# use_frameworks!
# Pods for PopUpDemo
pod 'SCLAlertView-Objective-C'
target 'PopUpDemoTests' do
inherit! :search_paths
# Pods for testing
end
target 'PopUpDemoUITests' do
inherit! :search_paths
# Pods for testing
end
end
<p dir="auto">After you write the code, go to the terminal and change directory to your project and run <code>pod install command to install the dependency. Then, open up the .xcworkspace to be able to use libraries in the Podfile.<br />
<img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517123791/wvmpja1yjktqgpvxb3sl.png" alt="Screen Shot 2018-01-26 at 6.23.33 PM.png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517123791/wvmpja1yjktqgpvxb3sl.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517123791/wvmpja1yjktqgpvxb3sl.png 2x" /><br />
<b>Importing the library to ViewController<br />
Go to your ViewController class, we will import SCLAlertView library and its style kit using these lines.
<pre><code>//
// ViewController.h
// PopUpDemo
//
// Created by Andri on 1/26/18.
// Copyright © 2018 Andri. All rights reserved.
//
#import <UIKit/UIKit.h>
#import "SCLAlertView.h"
#import "SCLAlertViewStyleKit.h"
@interface ViewController : UIViewController
@end
<p dir="auto">After done with the import library, we will use and implement it in our ViewController.m class.<br />
<b>Use and implement the SCLAlertView<br />
First of all, we'll initialize using common way like this line. We allocate using initWithNewWindow.<br />
<code>SCLAlertView *alert = [[SCLAlertView alloc] initWithNewWindow];<br />
When we have declared, we'll try to show success alert first. Write down this line.
<pre><code>[alert showSuccess:@"Alert Success" subTitle:@"This is a your success info to user." closeButtonTitle:@"Done" duration:0.0f];
<p dir="auto">We have some parameters above that consist of:
<ul>
<li><code>showSuccess this is a kind of alert type and also provide we to write message to the title
<li><code>subTitle this is the detail of the alert we give to the users.
<li><code>closeButtonTitle this is the text of our close button
<li><code>duration specify how long the popup is showing to the screen. If we are using 0.0f, then the popup will never disappear like we write above.<br />
When you run the code, you will see the result like this<br />
<img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517124476/klslbd8lkvdhmuvdj0u3.png" alt="Screen Shot 2018-01-28 at 2.27.24 PM.png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517124476/klslbd8lkvdhmuvdj0u3.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517124476/klslbd8lkvdhmuvdj0u3.png 2x" /><br />
Okay, let's continue with the showError. It's almost identical except the showError method. Write these lines to show error.
<pre><code>[alert showError:@"Alert Error" subTitle:@"This is a more descriptive error text." closeButtonTitle:@"OK" duration:0.0f];
<p dir="auto">And you will see the result like this.<br />
<img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517124757/w12qyigu4mtza48oggrc.png" alt="Screen Shot 2018-01-28 at 2.32.20 PM.png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517124757/w12qyigu4mtza48oggrc.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517124757/w12qyigu4mtza48oggrc.png 2x" /><br />
The same goes to showWarning method, write this code
<pre><code>[alert showWarning:@"Hello Warning" subTitle:@"This is a more descriptive warning text." closeButtonTitle:@"Done" duration:0.0f];
<p dir="auto">Run it, and you will see this.<br />
<img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517124995/nogv4oxp2ss1gq2vtwf8.png" alt="Screen Shot 2018-01-28 at 2.36.16 PM.png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517124995/nogv4oxp2ss1gq2vtwf8.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517124995/nogv4oxp2ss1gq2vtwf8.png 2x" /><br />
We can also custom the alert using custom image and color using showCustom method with some additional parameters like this.
<pre><code>[alert showCustom:[UIImage imageNamed:@"git"] color:[UIColor grayColor] title:@"Custom" subTitle:@"Add a custom icon and color for your own type of alert!" closeButtonTitle:@"OK" duration:0.0f];
<p dir="auto">The result will be like this<br />
<img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517125106/n45dnvxe0dguw3n9wopt.png" alt="Screen Shot 2018-01-28 at 2.37.55 PM.png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517125106/n45dnvxe0dguw3n9wopt.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517125106/n45dnvxe0dguw3n9wopt.png 2x" /><br />
You can try another type by exploring the method of each alert type. And if we combine some alert type like these lines.
<pre><code>
[alert showSuccess:@"Alert Success" subTitle:@"This is a your success info to user." closeButtonTitle:@"Done" duration:0.0f];
[alert showError:@"Alert Error" subTitle:@"This is a more descriptive error text." closeButtonTitle:@"OK" duration:0.0f];
[alert showNotice:@"Hello Notice" subTitle:@"This is a more descriptive notice text." closeButtonTitle:@"Done" duration:0.0f]; // Notice
[alert showWarning:@"Hello Warning" subTitle:@"This is a more descriptive warning text." closeButtonTitle:@"Done" duration:0.0f]; // Warning
[alert showInfo:@"Hello Info" subTitle:@"This is a more descriptive info text." closeButtonTitle:@"Done" duration:0.0f]; // Info
[alert showEdit:@"Hello Edit" subTitle:@"This is a more descriptive info text with a edit textbox" closeButtonTitle:@"Done" duration:0.0f]; // Edit
[alert showCustom:[UIImage imageNamed:@"git"] color:[UIColor grayColor] title:@"Custom" subTitle:@"Add a custom icon and color for your own type of alert!" closeButtonTitle:@"OK" duration:0.0f];
[alert showWaiting:@"Waiting..." subTitle:@"Blah de blah de blah, blah. Blah de blah de" closeButtonTitle:nil duration:0.0f];
[alert showQuestion:@"Question?" subTitle:@"SINI" closeButtonTitle:@"Dismiss" duration:0.0f];
<p dir="auto">we'll see some stackup alert like this.<br />
<img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517125482/y7kuwwmfsvwg7osc7lqx.png" alt="Screen Shot 2018-01-28 at 2.41.15 PM.png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517125482/y7kuwwmfsvwg7osc7lqx.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517125482/y7kuwwmfsvwg7osc7lqx.png 2x" /><br />
The next thing we are going to do is using the SCLAlertViewBuilder. This is the builder version to initialize and styling the builder. Write down this code.
<pre><code>SCLAlertViewBuilder *builder = [SCLAlertViewBuilder new]
.addButtonWithActionBlock(@"Send", ^{ /*work here*/ });
SCLAlertViewShowBuilder *showBuilder = [SCLAlertViewShowBuilder new]
.style(SCLAlertViewStyleWarning)
.title(@"Title")
.subTitle(@"Subtitle")
.duration(0);
[showBuilder showAlertView:builder.alertView onViewController:self];
<p dir="auto">We first initialize the builder, and then <code>addButtonWithActionBlock to be able to pass callback function when the action button is tapped. Then, we initialize the <code>SCLAlertViewShowBuilder and give it warning style, give title, subtitle and duration. Then we show it using <code>[showBuilder showAlertView:builder.alertView onViewController:self];. When you run the app, you will see something like this.<br />
<img src="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517125780/u8qgqcqmxp2wh6dyw1kf.png" alt="Screen Shot 2018-01-28 at 2.49.16 PM.png" srcset="https://images.hive.blog/768x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517125780/u8qgqcqmxp2wh6dyw1kf.png 1x, https://images.hive.blog/1536x0/https://res.cloudinary.com/hpiynhbhq/image/upload/v1517125780/u8qgqcqmxp2wh6dyw1kf.png 2x" /><br />
If you tap the send button the callback function will be called. Okay, that's all about this alert tutorial. Thanks for reading this guide.
<h4>Curriculum
<ul>
<li><span><a href="https://utopian.io/utopian-io/@andrixyz/creating-floating-action-button-in-ios-development-like-in-path-app" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">https://utopian.io/utopian-io/@andrixyz/creating-floating-action-button-in-ios-development-like-in-path-app
<li><span><a href="https://utopian.io/utopian-io/@andrixyz/working-with-realm-to-create-a-simple-to-do-list-ios-app-development" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">https://utopian.io/utopian-io/@andrixyz/working-with-realm-to-create-a-simple-to-do-list-ios-app-development
<p dir="auto"><br /><hr /><em>Posted on <a href="https://utopian.io/utopian-io/@andrixyz/how-to-display-pop-up-in-ios-development-using-sclalertview" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Utopian.io - Rewarding Open Source Contributors<hr /><p>
@andrixyz, Contribution to open source project, I like you and upvote.
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @andrixyz I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x