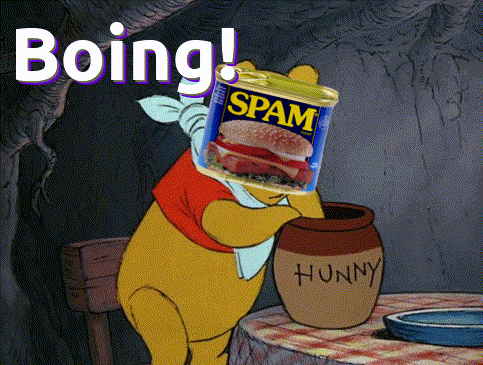
<p dir="auto">Have you wondered how you can make your own gifs?
<p dir="auto">Now, certainly, this is not the easiest way, but it is a fun project for learning, and heck, it might come in useful one day ;)
<p dir="auto"><a href="https://steemit.com/linux/@makerhacks/converting-videos-and-creating-timelapse-from-photographs-in-linux" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">You may remember that I already covered how to create a movie from time-lapse photographs, this is similar and takes the technique a couple of steps further.
<h3>Code
<h4><a href="https://gist.github.com/omiq/159e042339d3d27f2e6a490924d881fd" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">Full Code Gist Here
<p dir="auto">First, we need some modules.
<p dir="auto">OS and Shutil are for shell operations, because we need to call tools and create/delete folders.
<pre><code>import os
import shutil
from PIL import ImageFont
from PIL import Image
from PIL import ImageDraw
<p dir="auto">Next, we create the folder if it does not already exist, nuking the contents if it does.
<p dir="auto">We also create our image, loading in a background first.
<pre><code>if os.path.exists('frames'):
shutil.rmtree('frames')
os.mkdir('frames')
img_background = Image.open('background.png')
img = Image.new("RGBA", img_background.size, (0, 0, 0, 255))
<p dir="auto">We need three colours, and a nice font.
<pre><code>x = 10
y = 10
silver = (100, 100, 100, 255)
purple = (100, 0, 200, 255)
white = (255, 255, 255, 255)
text = '''Boing!'''
font = ImageFont.truetype("/home/chrisg/Ubuntu-B.ttf", 75)
draw = ImageDraw.Draw(img)
<p dir="auto">Now we create the frames of animation, 24 frames for 1 second. All I am doing here is adding text to each frame in a different location, you can get wild in yours!
<pre><code>for N in range(0, 24):
y += N
img.paste(img_background, (0, 0))
draw.text((x+4, y+4), text, purple, font=font)
draw.text((x+2, y+2), text, silver, font=font)
draw.text((x, y), text, white, font=font)
img.save("./frames/{}.png".format(str(N).zfill(3)))
<p dir="auto">All that is left is to generate an AVI then convert to gif.
<p dir="auto">For higher quality we can come back and <a href="https://steemit.com/programming/@makerhacks/how-to-get-higher-quality-gifs-with-ffmpeg" target="_blank" rel="noreferrer noopener" title="This link will take you away from hive.blog" class="external_link">optimize the palette, but for simplicity this is sufficient for now!
<pre><code>os.system('ffmpeg -framerate 24 -i frames/%03d.png -c:v ffv1 -r 24 -y out.avi')
os.system('ffmpeg -y -i out.avi out.gif')
shutil.rmtree('frames')
<hr /><span>
<img src="https://images.hive.blog/768x0/https://cdn.steemitimages.com/0x0/https://cdn.discordapp.com/attachments/383256479056134146/446022370608676864/makerhacks.png" srcset="https://images.hive.blog/768x0/https://cdn.steemitimages.com/0x0/https://cdn.discordapp.com/attachments/383256479056134146/446022370608676864/makerhacks.png 1x, https://images.hive.blog/1536x0/https://cdn.steemitimages.com/0x0/https://cdn.discordapp.com/attachments/383256479056134146/446022370608676864/makerhacks.png 2x" />
Awesome.
I've created animated gifs from the command line a few times. It might be a pain in the ass, but there's a lot that you can do surprisingly easily if you take the time to look up how. I've never really done it with python though. Always thought about creating a tool to help me make gifs, but never got around to it.
Perhaps I should mess around with doing some stuff and create a little library of useful bits of code for making little fun gifs. :)
Share when you do :D
Thanks for Lesson! I would Surely Try to Make it with python and share it with you 😊
I am still learning it. I am practicing it but never posted. It is very hard. I have to familiarize & understand the commands. Thanks for the post another info.