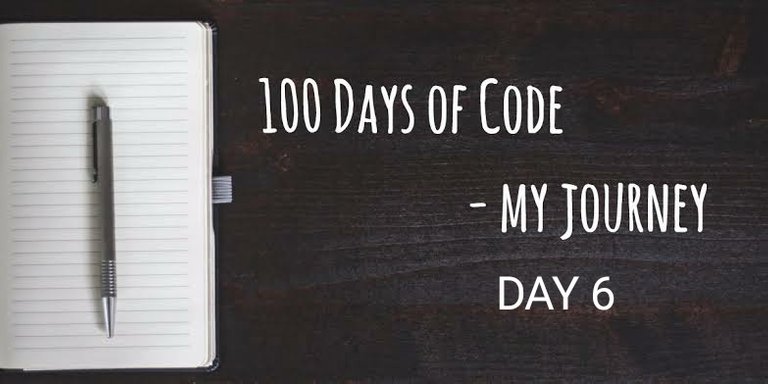
<p dir="auto">Problem: Caesars Cypher ROT13.
<p dir="auto">Problem Definition: Caesars Cypher ROT13 decodes word by shifting it thirteen places backward.
<p dir="auto">Algorithm
<ol>
<li><p dir="auto">The given string is splitted into an array/list of characters.
<li><p dir="auto">I looped through each character and get its ASCII code.
<li><p dir="auto">I then checked if each character ASCII code is between A-Z and return the character as it is if it's not between A-Z .
<li><p dir="auto">I shifted character code of character whose ASCII code fall between A-Z by 13 and return the corresponding character at that position.
<li><p dir="auto">For character with ASCII code lesser than 78, I shifted them forward by 13 places so as to fall between A-Z .
<p dir="auto">JavaScript Code
<p dir="auto">function ROT13(str) {
<p dir="auto">return str.split(" ").map.call(str, function(char){<br />
x = char.charCodeAt(0);
<pre><code> if (x < 65 || x > 90) {
return String.fromCharCode(x);
}
else if (x < 78) {
return String.fromCharCode(x + 13);
}
return String.fromCharCode(x - 13);
<p dir="auto">}).join(" ");
<p dir="auto">}